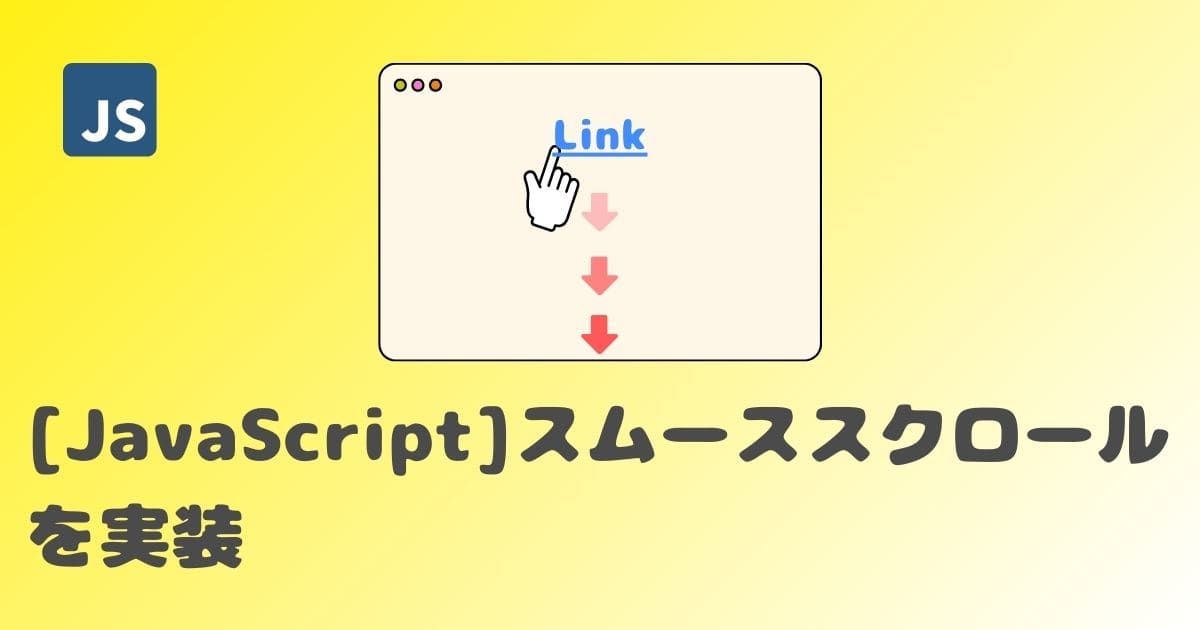
【JavaScript】input type=”date”の日付フォーマットを変更する方法を解説
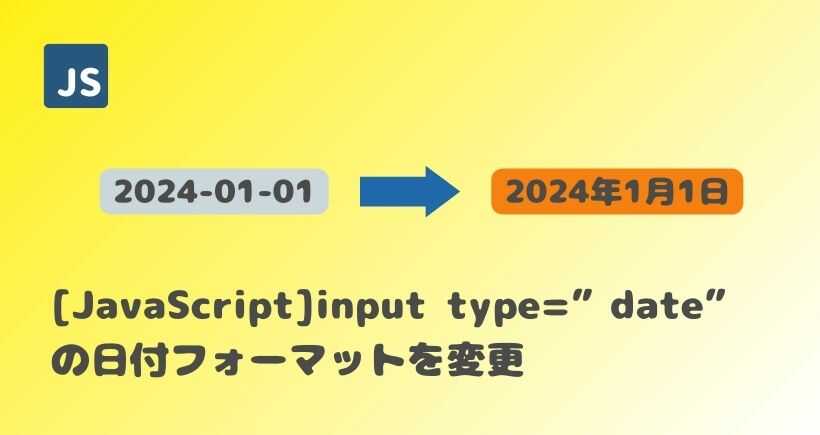
input type=”date”(日付入力フォーム)に入力した日付をJavaScriptを使って表示させると「2024-01-01」となります。ですが「2024年1月1日」や「2024/01/01」など別の日付フォーマットに変えるにはどうしたらよいのでしょうか?
今回はinput type=”date”の日付フォーマットを変更する方法をご紹介します。この記事では以下のお悩みを解決します。
- input type=”date”(日付入力フォーム)の日付フォーマットを変えたい
1. input type=”date”のデフォルトの日付フォーマット
最初のところでも述べたようにinput type=”date”の日付フォーマットは2024年1月1日の場合「2024-01-01」と表示されます。まずは実際に日付をフォームに入力して表示させてフォーマットを確認しましょう。
以下のコードはinput type=”date”に日付を入力し、「表示する」を押すと入力した日付が表示されます。以下のCodePenの「Run Pen」を押して実際に日付を入力して表示されるか確認してみてください。
See the Pen Untitled by Hiro (@Coffee1610) on CodePen.
<div class="wrapper">
<input type="date" class="input-date">
<button class="btn">表示する</button>
<p class="display"></p>
</div>
HTMLの解説
2行目: <input type=”date” class=”input-date”>
日付を入力するフォームです。
3行目:<button class=”btn”>表示する</button>
日付を入力した状態でこのボタンを押すと日付が表示されます。
4行目: <p class=”display”></p>
日付を表示させるためのタグで中身は空です。
//inputフォームを取得
const inputDate = document.querySelector('.input-date');
//ボタンを取得
const btn = document.querySelector('.btn');
//日付を表示するためのpタグを取得
const display = document.querySelector('.display');
//ボタンをクリックすると処理を実行
btn.addEventListener('click', () => {
//日付を選択していないときは処理が終了
if(!inputDate.value) {
return;
}
//pタグに日付を代入
display.innerText = '入力した日付: ' + inputDate.value;
});
JavaScriptの解説
2行目:const inputDate = document.querySelector(‘.input-date’);
日付入力フォーム(HTMLの2行目)を取得しています。
4行目:const btn = document.querySelector(‘.btn’);
ボタン(HTMLの3行目)を取得しています。
6行目:const display = document.querySelector(‘.display’);
日付を表示するためのpタグ(HTMLの4行目)を取得しています。
9-16行目:btn.addEventListener(‘click’, () => {~});
「表示する」ボタンをクリックすると処理を実行します。
11-13行目:if(!inputDate.value) {return;}
日付が空(選択していない)の場合は処理を終了します。inputDate.valueには選択した日付が入っています。
15行目:display.innerText = ‘入力した日付: ’ + inputDate.value;
pタグに取得した日付を書き込みます。「日付: 2024-01-01」のように表示されます。
2. 日付のフォーマットを変更する
次に入力した日付フォーマットを変更する方法をご紹介します。
2-1. 「2024/1/1」の形式で表示
2024年の1月1日を指定した場合「2024/1/1」に表示するようにします。以下のCodePenの「RunPen」を押して実際に入力して確認してみてください。
See the Pen 日付表示2 by Hiro (@Coffee1610) on CodePen.
<div class="wrapper">
<input type="date" class="input-date">
<button class="btn">表示する</button>
<p class="display"></p>
</div>
//inputフォームを取得
const inputDate = document.querySelector('.input-date');
//ボタンを取得
const btn = document.querySelector('.btn');
//日付を表示するためのpタグを取得
const display = document.querySelector('.display');
//ボタンをクリックすると処理を実行
btn.addEventListener('click', () => {
//日付を選択していないときは処理が終了
if(!inputDate.value) {
return;
}
//日付を取得
const fullDate = new Date(inputDate.value);
//年を取得
const getYear = fullDate.getFullYear();
//月を取得
const getMonth = fullDate.getMonth()+1;
//日を取得
const getDate = fullDate.getDate();
// 日付を2024/1/1の形式にする
const formattedDate = `${getYear}/${getMonth}/${getDate}`;
//pタグに日付を代入
display.innerText = '入力した日付: ' + formattedDate;
});
JavaScriptの解説
16行目:const fullDate = new Date(inputDate.value);
newDate()は現在、または指定した日時を表すためのオブジェクトです。newDate()を使うことで日付フォーマットを変更するためにJavaScriptで扱いやすい形式に変換することができます。変換後はJavaScript内で年、月、日を個別に取得できるようになり、フォーマットの変更もできるようになります。
18行目:const getYear = fullDate.getFullYear();
getFullYear()は指定した「年」(2024のような4桁の数字)を返します。
20行目:const getMonth = fullDate.getMonth()+1;
getMonth()は指定した「月」(「0-11」までの数値)を返します。例えば1月ならgetMonth()は「0」を返すので1を足すことで変数getMonthは「1」になります。
22行目:const getDate = fullDate.getDate();
getDate()は指定した「日」(「1-31」 までの数値)を返します。
25行目:const formattedDate = `${getYear}/${getMonth}/${getDate}`
;
日付のフォーマットを指定します(例:2024/1/1)。変数はテンプレートリテラル${~}で表示します。
2-2. 「2024/01/01」月日を0埋めする形式で表示する
月と日が1桁の場合、0埋めをして表示するようにします。2024年の1月1日を指定した場合「2024/01/01」に表示するようにします。以下のCodePenの「RunPen」を押して実際に入力して確認してみてください。
See the Pen 日付表示3 by Hiro (@Coffee1610) on CodePen.
<div class="wrapper">
<input type="date" class="input-date">
<button class="btn">表示する</button>
<p class="display"></p>
</div>
//inputフォームを取得
const inputDate = document.querySelector('.input-date');
//ボタンを取得
const btn = document.querySelector('.btn');
//日付を表示するためのpタグを取得
const display = document.querySelector('.display');
//ボタンをクリックすると処理を実行
btn.addEventListener('click', () => {
//日付を選択していないときは処理が終了
if(!inputDate.value) {
return;
}
//日付を取得
const fullDate = new Date(inputDate.value);
//年を取得
const getYear = fullDate.getFullYear();
//月を取得
const getMonth = fullDate.getMonth()+1;
//日を取得
const getDate = fullDate.getDate();
//日付を「2024/01/01」の形式にする 1桁の月日を0埋めにして表示する
const formattedDate = `${getYear}/${('0' + getMonth).slice(-2)}/${('0' + getDate).slice(-2)}`;
//pタグに日付を代入
display.innerText = '入力した日付: ' + formattedDate;
});
JavaScriptの解説
26行目:const formattedDate = `${getYear}/${('0' + getMonth).slice(-2)}/${('0' + getDate).slice(-2)}
`;
日付のフォーマットを指定しています。slice()は指定した文字数を文字列から切り取ります。マイナスの値が指定されている場合は文字列の後ろから切り取ります。slice(-2)を指定しているので文字列の後ろから2文字切り取ります。変数はテンプレートリテラル${~}で表示します。
例えば、1月や1日の場合「01」になり後ろから2文字切り取るのでそのまま「01」になり、1桁の月日でも2桁で表示されます。12月や12日の場合「012」になり後ろから2文字切り取るので「12」になります。
2-3. 「2024年1月1日(月)」の形式で表示
2024年1月1日を入力する場合「2024年1月1日(月)」と日付と曜日を表示するようにします。以下のCodePenの「RunPen」を押して実際に入力して確認してみてください。
See the Pen 日付表示4 by Hiro (@Coffee1610) on CodePen.
<div class="wrapper">
<input type="date" class="input-date">
<button class="btn">表示する</button>
<p class="display"></p>
</div>
//inputフォームを取得
const inputDate = document.querySelector('.input-date');
//ボタンを取得
const btn = document.querySelector('.btn');
//日付を表示するためのpタグを取得
const display = document.querySelector('.display');
//ボタンをクリックすると処理を実行
btn.addEventListener('click', () => {
//日付を選択していないときは処理が終了
if(!inputDate.value) {
return;
}
//日付を取得
const fullDate = new Date(inputDate.value);
//年を取得
const getYear = fullDate.getFullYear();
//月を取得
const getMonth = fullDate.getMonth()+1;
//日を取得
const getDate = fullDate.getDate();
//曜日を取得
const getDays = fullDate.getDay();
//曜日を表示したい形式で配列に入れる
const daysOfWeek = ['日','月','火','水','木','金','土'];
//配列から曜日を取り出す
const dayOfWeek = daysOfWeek[getDays];
//日付を「2024年1月1日(月)」のフォーマットにする
const formattedDate = `${getYear}年${getMonth}月${getDate}日(${dayOfWeek})`;
//pタグに日付を代入
display.innerText = '入力した日付: ' + formattedDate;
});
JavaScriptの解説
24行目:const getDays = fullDate.getDay();
getDay()は指定した「曜日」(「0-6」までの値)を返します。「日」は0、「月」は1、「火」は2…のように続きます。
26行目:const daysOfWeek = [‘日’,’月’,’火’,’水’,’木’,’金’,’土’];
曜日を配列に入れています。曜日はこの配列から取り出して表示されるので日曜日なら「日」と表示されます。例えば曜日を英語で表示したいような場合はconst daysOfWeek =[‘Sun’,’Mon’,’Tue’,’Wed’,’Thu’,’Fri’,’Sat’];のように指定します。
28行目:dayOfWeek = daysOfWeek[getDays];
指定した曜日を26行目の配列から取り出します。
31行目:const formattedDate = `${getYear}年${getMonth}月${getDate}日(${dayOfWeek})`
;
日付のフォーマット(例:2024年1月1日(月))を指定します。変数はテンプレートリテラル${~}で表示します。
3. まとめ
今回はinput type=”date”の日付フォーマットを変更する方法をご紹介しました。JavaScriptを使えば柔軟に日付フォーマットを変更できますので参考にしていただければ幸いです。